DESIGN PATTERN GUIDE -4TH PART
Observer Pattern
Observer pattern is used when there is one to many relationship between objects such as if one object is modified, its depenedent objects are to be notified automatically. Observer pattern falls under behavioral pattern category.
Implementation
Observer pattern uses three actor classes. Subject, Observer and Client. Subject, an object having methods to attach and de-attach observers to a client object. We've created classes Subject, Observerabstract class and concrete classes extending the abstract class the Observer.
ObserverPatternDemo, our demo class will use Subject and concrete class objects to show observer pattern in action.
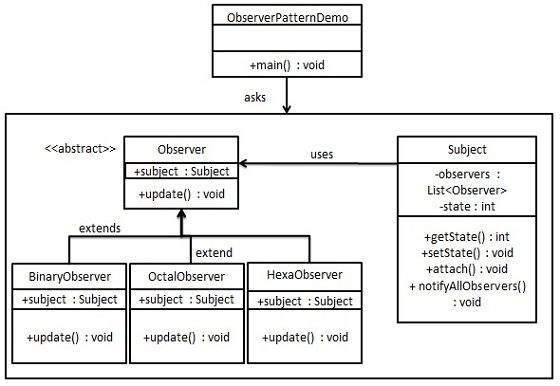
Step 1
Create Subject class.
Subject.java
import java.util.ArrayList; import java.util.List; public class Subject { private List<Observer> observers = new ArrayList<Observer>(); private int state; public int getState() { return state; } public void setState(int state) { this.state = state; notifyAllObservers(); } public void attach(Observer observer){ observers.add(observer); } public void notifyAllObservers(){ for (Observer observer : observers) { observer.update(); } } }
Step 2
Create Observer class.
Observer.java
public abstract class Observer { protected Subject subject; public abstract void update(); }
Step 3
Create concrete observer classes
BinaryObserver.java
public class BinaryObserver extends Observer{ public BinaryObserver(Subject subject){ this.subject = subject; this.subject.attach(this); } @Override public void update() { System.out.println( "Binary String: " + Integer.toBinaryString( subject.getState() ) ); } }
OctalObserver.java
public class OctalObserver extends Observer{ public OctalObserver(Subject subject){ this.subject = subject; this.subject.attach(this); } @Override public void update() { System.out.println( "Octal String: " + Integer.toOctalString( subject.getState() ) ); } }
HexaObserver.java
public class HexaObserver extends Observer{ public HexaObserver(Subject subject){ this.subject = subject; this.subject.attach(this); } @Override public void update() { System.out.println( "Hex String: " + Integer.toHexString( subject.getState() ).toUpperCase() ); } }
Step 4
Use Subject and concrete observer objects.
ObserverPatternDemo.java
public class ObserverPatternDemo { public static void main(String[] args) { Subject subject = new Subject(); new HexaObserver(subject); new OctalObserver(subject); new BinaryObserver(subject); System.out.println("First state change: 15"); subject.setState(15); System.out.println("Second state change: 10"); subject.setState(10); } }
Step 5
Verify the output.
First state change: 15 Hex String: F Octal String: 17 Binary String: 1111 Second state change: 10 Hex String: A Octal String: 12 Binary String: 1010
State Pattern
In State pattern a class behavior changes based on its state. This type of design pattern comes under behavior pattern.
In State pattern, we create objects which represent various states and a context object whose behavior varies as its state object changes.
Implementation
We're going to create a State interface defining a action and concrete state classes implementing theState interface. Context is a class which carries a State.
StaePatternDemo, our demo class will use Context and state objects to demonstrate change in Context behavior based on type of state it is in.

Step 1
Create an interface.
Image.java
public interface State { public void doAction(Context context); }
Step 2
Create concrete classes implementing the same interface.
StartState.java
public class StartState implements State { public void doAction(Context context) { System.out.println("Player is in start state"); context.setState(this); } public String toString(){ return "Start State"; } }
StopState.java
public class StopState implements State { public void doAction(Context context) { System.out.println("Player is in stop state"); context.setState(this); } public String toString(){ return "Stop State"; } }
Step 3
Create Context Class.
Context.java
public class Context { private State state; public Context(){ state = null; } public void setState(State state){ this.state = state; } public State getState(){ return state; } }
Step 4
Use the Context to see change in behaviour when State changes.
StatePatternDemo.java
public class StatePatternDemo { public static void main(String[] args) { Context context = new Context(); StartState startState = new StartState(); startState.doAction(context); System.out.println(context.getState().toString()); StopState stopState = new StopState(); stopState.doAction(context); System.out.println(context.getState().toString()); } }
Step 5
Verify the output.
Player is in start state Start State Player is in stop state Stop State
Null Object Pattern
In Null Object pattern, a null object replaces check of NULL object instance. Instead of putting if check for a null value, Null Object reflects a do nothing relationship. Such Null object can also be used to provide default behaviour in case data is not available.
In Null Object pattern, we create a abstract class specifying the various operations to be done, concreate classes extending this class and a null object class providing do nothing implemention of this class and will be used seemlessly where we need to check null value.
Implementation
We're going to create a AbstractCustomer abstract class defining opearations, here the name of the customer and concrete classes extending the AbstractCustomer class. A factory classCustomerFactory is created to return either RealCustomer or NullCustomer objects based on the name of customer passed to it.
NullPatternDemo, our demo class will use CustomerFactory to demonstrate use of Null Object pattern.
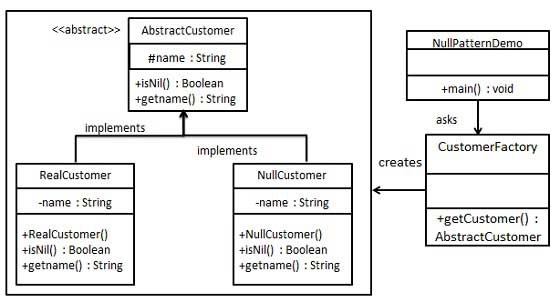
Step 1
Create an abstract class.
AbstractCustomer.java
public abstract class AbstractCustomer { protected String name; public abstract boolean isNil(); public abstract String getName(); }
Step 2
Create concrete classes extending the above class.
RealCustomer.java
public class RealCustomer extends AbstractCustomer { public RealCustomer(String name) { this.name = name; } @Override public String getName() { return name; } @Override public boolean isNil() { return false; } }
NullCustomer.java
public class NullCustomer extends AbstractCustomer { @Override public String getName() { return "Not Available in Customer Database"; } @Override public boolean isNil() { return true; } }
Step 3
Create CustomerFactory Class.
CustomerFactory.java
public class CustomerFactory { public static final String[] names = {"Rob", "Joe", "Julie"}; public static AbstractCustomer getCustomer(String name){ for (int i = 0; i < names.length; i++) { if (names[i].equalsIgnoreCase(name)){ return new RealCustomer(name); } } return new NullCustomer(); } }
Step 4
Use the CustomerFactory get either RealCustomer or NullCustomer objects based on the name of customer passed to it.
NullPatternDemo.java
public class NullPatternDemo { public static void main(String[] args) { AbstractCustomer customer1 = CustomerFactory.getCustomer("Rob"); AbstractCustomer customer2 = CustomerFactory.getCustomer("Bob"); AbstractCustomer customer3 = CustomerFactory.getCustomer("Julie"); AbstractCustomer customer4 = CustomerFactory.getCustomer("Laura"); System.out.println("Customers"); System.out.println(customer1.getName()); System.out.println(customer2.getName()); System.out.println(customer3.getName()); System.out.println(customer4.getName()); } }
Step 5
Verify the output.
Customers Rob Not Available in Customer Database Julie Not Available in Customer Database
Strategy Pattern
In Strategy pattern, a class behavior or its algorithm can be changed at run time. This type of design pattern comes under behavior pattern.
In Strategy pattern, we create objects which represent various strategies and a context object whose behavior varies as per its strategy object. The strategy object changes the executing algorithm of the context object.
Implementation
We're going to create a Strategy interface defining a action and concrete strategy classes implementing the Strategy interface. Context is a class which uses a Strategy.
StrategyPatternDemo, our demo class will use Context and strategy objects to demonstrate change in Context behaviour based on strategy it deploys or uses.
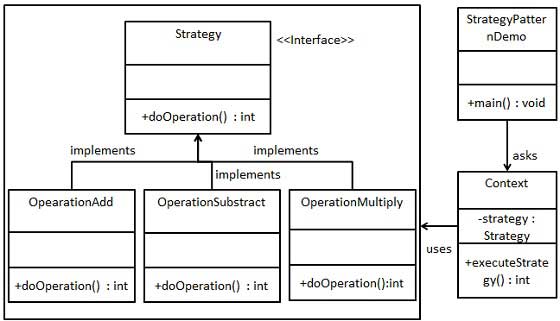
Step 1
Create an interface.
Strategy.java
public interface Strategy { public int doOperation(int num1, int num2); }
Step 2
Create concrete classes implementing the same interface.
OperationAdd.java
public class OperationAdd implements Strategy{ @Override public int doOperation(int num1, int num2) { return num1 + num2; } }
OperationSubstract.java
public class OperationSubstract implements Strategy{ @Override public int doOperation(int num1, int num2) { return num1 - num2; } }
OperationMultiply.java
public class OperationMultiply implements Strategy{ @Override public int doOperation(int num1, int num2) { return num1 * num2; } }
Step 3
Create Context Class.
Context.java
public class Context { private Strategy strategy; public Context(Strategy strategy){ this.strategy = strategy; } public int executeStrategy(int num1, int num2){ return strategy.doOperation(num1, num2); } }
Step 4
Use the Context to see change in behaviour when it changes its Strategy.
StatePatternDemo.java
public class StrategyPatternDemo { public static void main(String[] args) { Context context = new Context(new OperationAdd()); System.out.println("10 + 5 = " + context.executeStrategy(10, 5)); context = new Context(new OperationSubstract()); System.out.println("10 - 5 = " + context.executeStrategy(10, 5)); context = new Context(new OperationMultiply()); System.out.println("10 * 5 = " + context.executeStrategy(10, 5)); } }
Step 5
Verify the output.
10 + 5 = 15 10 - 5 = 5 10 * 5 = 50
Template Pattern
In Template pattern, an abstract class exposes defined way(s)/template(s) to execute its methods. Its subclasses can overrides the method implementations as per need basis but the invocation is to be in the same way as defined by an abstract class. This pattern comes under behavior pattern category.
Implementation
We're going to create a Game abstract class defining operations with a template method set to be final so that it cannot be overridden. Cricket and Football are concrete classes extend Game and override its methods.
TemplatePatternDemo, our demo class will use Game to demonstrate use of template pattern.
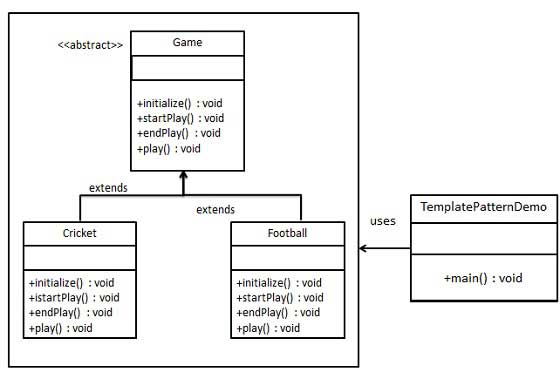
Step 1
Create an abstract class with a template method being final.
Game.java
public abstract class Game { abstract void initialize(); abstract void startPlay(); abstract void endPlay(); //template method public final void play(){ //initialize the game initialize(); //start game startPlay(); //end game endPlay(); } }
Step 2
Create concrete classes extending the above class.
Cricket.java
public class Cricket extends Game { @Override void endPlay() { System.out.println("Cricket Game Finished!"); } @Override void initialize() { System.out.println("Cricket Game Initialized! Start playing."); } @Override void startPlay() { System.out.println("Cricket Game Started. Enjoy the game!"); } }
Football.java
public class Football extends Game { @Override void endPlay() { System.out.println("Football Game Finished!"); } @Override void initialize() { System.out.println("Football Game Initialized! Start playing."); } @Override void startPlay() { System.out.println("Football Game Started. Enjoy the game!"); } }
Step 3
Use the Game's template method play() to demonstrate a defined way of playing game.
TemplatePatternDemo.java
public class TemplatePatternDemo { public static void main(String[] args) { Game game = new Cricket(); game.play(); System.out.println(); game = new Football(); game.play(); } }
Step 4
Verify the output.
Cricket Game Initialized! Start playing. Cricket Game Started. Enjoy the game! Cricket Game Finished! Football Game Initialized! Start playing. Football Game Started. Enjoy the game! Football Game Finished!
Visitor Pattern
In Visitor pattern, we use a visitor class which changes the executing algorithm of an element class. By this way, execution algorithm of element can varies as visitor varies. This pattern comes under behavior pattern category. As per the pattern, element object has to accept the visitor object so that visitor object handles the operation on the element object.
Implementation
We're going to create a ComputerPart interface defining accept opearation.Keyboard, Mouse, Monitorand Computer are concrete classes implementing ComputerPart interface. We'll define another interface ComputerPartVisitor which will define a visitor class operations. Computer uses concrete visitor to do corresponding action.
VisitorPatternDemo, our demo class will use Computer, ComputerPartVisitor classes to demonstrate use of visitor pattern.
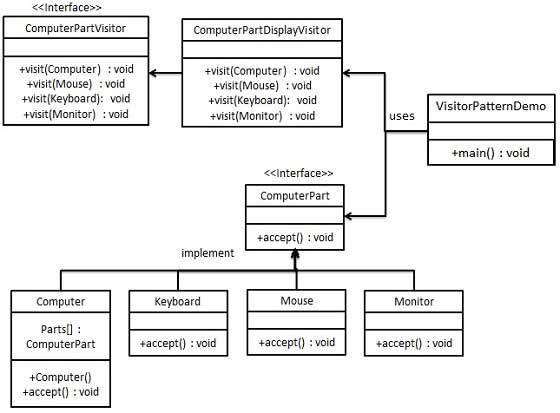
Step 1
Define an interface to represent element.
ComputerPart.java
public interface class ComputerPart { public void accept(ComputerPartVisitor computerPartVisitor); }
Step 2
Create concrete classes extending the above class.
Keyboard.java
public class Keyboard implements ComputerPart { @Override public void accept(ComputerPartVisitor computerPartVisitor) { computerPartVisitor.visit(this); } }
Monitor.java
public class Monitor implements ComputerPart { @Override public void accept(ComputerPartVisitor computerPartVisitor) { computerPartVisitor.visit(this); } }
Mouse.java
public class Mouse implements ComputerPart { @Override public void accept(ComputerPartVisitor computerPartVisitor) { computerPartVisitor.visit(this); } }
Computer.java
public class Computer implements ComputerPart { ComputerPart[] parts; public Computer(){ parts = new ComputerPart[] {new Mouse(), new Keyboard(), new Monitor()}; } @Override public void accept(ComputerPartVisitor computerPartVisitor) { for (int i = 0; i < parts.length; i++) { parts[i].accept(computerPartVisitor); } computerPartVisitor.visit(this); } }
Step 3
Define an interface to represent visitor.
ComputerPartVisitor.java
public interface ComputerPartVisitor { public void visit(Computer computer); public void visit(Mouse mouse); public void visit(Keyboard keyboard); public void visit(Monitor monitor); }
Step 4
Create concrete visitor implementing the above class.
ComputerPartDisplayVisitor.java
public class ComputerPartDisplayVisitor implements ComputerPartVisitor { @Override public void visit(Computer computer) { System.out.println("Displaying Computer."); } @Override public void visit(Mouse mouse) { System.out.println("Displaying Mouse."); } @Override public void visit(Keyboard keyboard) { System.out.println("Displaying Keyboard."); } @Override public void visit(Monitor monitor) { System.out.println("Displaying Monitor."); } }
Step 5
Use the ComputerPartDisplayVisitor to display parts of Computer.
VisitorPatternDemo.java
public class VisitorPatternDemo { public static void main(String[] args) { ComputerPart computer = new Computer(); computer.accept(new ComputerPartDisplayVisitor()); } }
Step 6
Verify the output.
Displaying Mouse. Displaying Keyboard. Displaying Monitor. Displaying Computer.
MVC Pattern
MVC Pattern stands for Model-View-Controller Pattern. This pattern is used to separate application's concerns.
- Model - Model represents an object or JAVA POJO carrying data. It can also have logic to update controller if its data changes.
- View - View represents the visualization of the data that model contains.
- Controller - Controller acts on both Model and view. It controls the data flow into model object and updates the view whenever data changes. It keeps View and Model separate.
Implementation
We're going to create a Student object acting as a model.StudentView will be a view class which can print student details on console and StudentController is the controller class responsible to store data in Student object and update view StudentView accordingly.
MVCPatternDemo, our demo class will use StudentController to demonstrate use of MVC pattern.
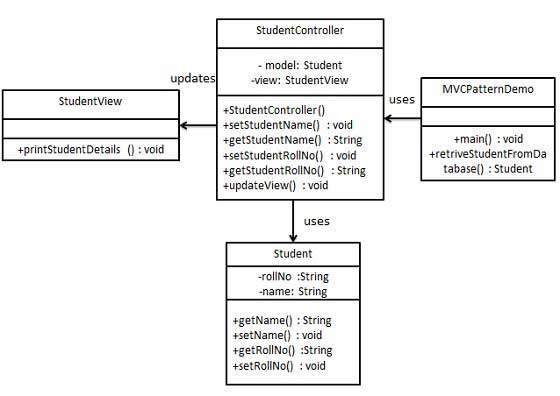
Step 1
Create Model.
Student.java
public class Student { private String rollNo; private String name; public String getRollNo() { return rollNo; } public void setRollNo(String rollNo) { this.rollNo = rollNo; } public String getName() { return name; } public void setName(String name) { this.name = name; } }
Step 2
Create View.
StudentView.java
public class StudentView { public void printStudentDetails(String studentName, String studentRollNo){ System.out.println("Student: "); System.out.println("Name: " + studentName); System.out.println("Roll No: " + studentRollNo); } }
Step 3
Create Controller.
StudentController.java
public class StudentController { private Student model; private StudentView view; public StudentController(Student model, StudentView view){ this.model = model; this.view = view; } public void setStudentName(String name){ model.setName(name); } public String getStudentName(){ return model.getName(); } public void setStudentRollNo(String rollNo){ model.setRollNo(rollNo); } public String getStudentRollNo(){ return model.getRollNo(); } public void updateView(){ view.printStudentDetails(model.getName(), model.getRollNo()); } }
Step 4
Use the StudentController methods to demonstrate MVC design pattern usage.
MVCPatternDemo.java
public class MVCPatternDemo { public static void main(String[] args) { //fetch student record based on his roll no from the database Student model = retriveStudentFromDatabase(); //Create a view : to write student details on console StudentView view = new StudentView(); StudentController controller = new StudentController(model, view); controller.updateView(); //update model data controller.setStudentName("John"); controller.updateView(); } private static Student retriveStudentFromDatabase(){ Student student = new Student(); student.setName("Robert"); student.setRollNo("10"); return student; } }
Step 5
Verify the output.
Student: Name: Robert Roll No: 10 Student: Name: Julie Roll No: 10
Business Delegate Pattern
Business Delegate Pattern is used to decouple presentation tier and business tier. It is basically use to reduce communication or remote lookup functionality to business tier code in presentation tier code. In business tier we've following entities.
- Client - Presentation tier code may be JSP, servlet or UI java code.
- Business Delegate - A single entry point class for client entities to provide access to Business Service methods.
- LookUp Service - Lookup service object is responsible to get relative business implementation and provide business object access to business delegate object.
- Business Service - Business Service interface. Concrete classes implements this business service to provide actual business implementation logic.
Implementation
We're going to create a Client, BusinessDelegate, BusinessService, LookUpService, JMSService andEJBService representing various entities of Business Delegate pattern.
BusinessDelegatePatternDemo, our demo class will use BusinessDelegate and Client to demonstrate use of Business Delegate pattern.
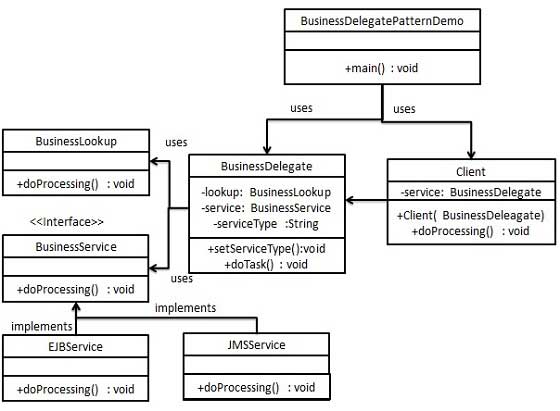
Step 1
Create BusinessService Interface.
BusinessService.java
public interface BusinessService { public void doProcessing(); }
Step 2
Create Concreate Service Classes.
EJBService.java
public class EJBService implements BusinessService { @Override public void doProcessing() { System.out.println("Processing task by invoking EJB Service"); } }
JMSService.java
public class JMSService implements BusinessService { @Override public void doProcessing() { System.out.println("Processing task by invoking JMS Service"); } }
Step 3
Create Business Lookup Service.
BusinessLookUp.java
public class BusinessLookUp { public BusinessService getBusinessService(String serviceType){ if(serviceType.equalsIgnoreCase("EJB")){ return new EJBService(); }else { return new JMSService(); } } }
Step 4
Create Business Delegate.
BusinessLookUp.java
public class BusinessDelegate { private BusinessLookUp lookupService = new BusinessLookUp(); private BusinessService businessService; private String serviceType; public void setServiceType(String serviceType){ this.serviceType = serviceType; } public void doTask(){ businessService = lookupService.getBusinessService(serviceType); businessService.doProcessing(); } }
Step 5
Create Client.
Student.java
public class Client { BusinessDelegate businessService; public Client(BusinessDelegate businessService){ this.businessService = businessService; } public void doTask(){ businessService.doTask(); } }
Step 6
Use BusinessDelegate and Client classes to demonstrate Business Delegate pattern.
BusinessDelegatePatternDemo.java
public class BusinessDelegatePatternDemo { public static void main(String[] args) { BusinessDelegate businessDelegate = new BusinessDelegate(); businessDelegate.setServiceType("EJB"); Client client = new Client(businessDelegate); client.doTask(); businessDelegate.setServiceType("JMS"); client.doTask(); } }
Step 7
Verify the output.
Processing task by invoking EJB Service Processing task by invoking JMS Service
No comments:
Post a Comment