DESIGN PATTERN GUIDE -5TH PART
Composite Entity Pattern
Composite Entity pattern is used in EJB persistence mechanism. A Composite entity is an EJB entity bean which represents a graph of objects. When a composite entity is updated, internally dependent objects beans get updated automatically as being managed by EJB entity bean. Following are the participants in Composite Entity Bean.
- Composite Entity - It is primary entity bean.It can be coarse grained or can contain a coarse grained object to be used for persistence purpose.
- Coarse-Grained Object -This object contains dependent objects. It has its own life cycle and also manages life cycle of dependent objects.
- Dependent Object - Dependent objects is an object which depends on Coarse-Grained object for its persistence lifecycle.
- Strategies - Strategies represents how to implement a Composite Entity.
Implementation
We're going to create CompositeEntity object acting as CompositeEntity. CoarseGrainedObject will be a class which contains dependent objects. CompositeEntityPatternDemo, our demo class will useClient class to demonstrate use of Composite Entity pattern.
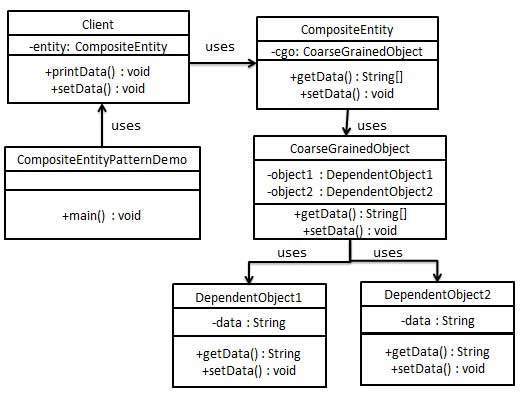
Step 1
Create Dependent Objects.
DependentObject1.java
public class DependentObject1 { private String data; public void setData(String data){ this.data = data; } public String getData(){ return data; } }
DependentObject2.java
public class DependentObject2 { private String data; public void setData(String data){ this.data = data; } public String getData(){ return data; } }
Step 2
Create Coarse Grained Object.
CoarseGrainedObject.java
public class CoarseGrainedObject { DependentObject1 do1 = new DependentObject1(); DependentObject2 do2 = new DependentObject2(); public void setData(String data1, String data2){ do1.setData(data1); do2.setData(data2); } public String[] getData(){ return new String[] {do1.getData(),do2.getData()}; } }
Step 3
Create Composite Entity.
CompositeEntity.java
public class CompositeEntity { private CoarseGrainedObject cgo = new CoarseGrainedObject(); public void setData(String data1, String data2){ cgo.setData(data1, data2); } public String[] getData(){ return cgo.getData(); } }
Step 4
Create Client class to use Composite Entity.
Client.java
public class Client { private CompositeEntity compositeEntity = new CompositeEntity(); public void printData(){ for (int i = 0; i < compositeEntity.getData().length; i++) { System.out.println("Data: " + compositeEntity.getData()[i]); } } public void setData(String data1, String data2){ compositeEntity.setData(data1, data2); } }
Step 5
Use the Client to demonstrate Composite Entity design pattern usage.
CompositeEntityPatternDemo.java
public class CompositeEntityPatternDemo { public static void main(String[] args) { Client client = new Client(); client.setData("Test", "Data"); client.printData(); client.setData("Second Test", "Data1"); client.printData(); } }
Step 6
Verify the output.
Data: Test Data: Data Data: Second Test Data: Data1
Data Access Object Pattern
Data Access Object Pattern or DAO pattern is used to separate low level data accessing API or operations from high level business services. Following are the participants in Data Access Object Pattern.
- Data Access Object Interface - This interface defines the standard operations to be performed on a model object(s).
- Data Access Object concrete class -This class implements above interface. This class is responsible to get data from a datasource which can be database / xml or any other storage mechanism.
- Model Object or Value Object - This object is simple POJO containing get/set methods to store data retrieved using DAO class.
Implementation
We're going to create a Student object acting as a Model or Value Object.StudentDao is Data Access Object Interface.StudentDaoImpl is concrete class implementing Data Access Object Interface.DaoPatternDemo, our demo class will use StudentDao demonstrate use of Data Access Object pattern.
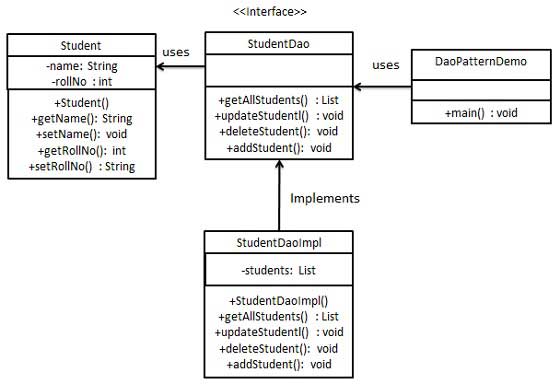
Step 1
Create Value Object.
Student.java
public class Student { private String name; private int rollNo; Student(String name, int rollNo){ this.name = name; this.rollNo = rollNo; } public String getName() { return name; } public void setName(String name) { this.name = name; } public int getRollNo() { return rollNo; } public void setRollNo(int rollNo) { this.rollNo = rollNo; } }
Step 2
Create Data Access Object Interface.
StudentDao.java
import java.util.List; public interface StudentDao { public List<Student> getAllStudents(); public Student getStudent(int rollNo); public void updateStudent(Student student); public void deleteStudent(Student student); }
Step 3
Create concreate class implementing above interface.
StudentDaoImpl.java
import java.util.ArrayList; import java.util.List; public class StudentDaoImpl implements StudentDao { //list is working as a database List<Student> students; public StudentDaoImpl(){ students = new ArrayList<Student>(); Student student1 = new Student("Robert",0); Student student2 = new Student("John",1); students.add(student1); students.add(student2); } @Override public void deleteStudent(Student student) { students.remove(student.getRollNo()); System.out.println("Student: Roll No " + student.getRollNo() +", deleted from database"); } //retrive list of students from the database @Override public List<Student> getAllStudents() { return students; } @Override public Student getStudent(int rollNo) { return students.get(rollNo); } @Override public void updateStudent(Student student) { students.get(student.getRollNo()).setName(student.getName()); System.out.println("Student: Roll No " + student.getRollNo() +", updated in the database"); } }
Step 4
Use the StudentDao to demonstrate Data Access Object pattern usage.
CompositeEntityPatternDemo.java
public class DaoPatternDemo { public static void main(String[] args) { StudentDao studentDao = new StudentDaoImpl(); //print all students for (Student student : studentDao.getAllStudents()) { System.out.println("Student: [RollNo : " +student.getRollNo()+", Name : "+student.getName()+" ]"); } //update student Student student =studentDao.getAllStudents().get(0); student.setName("Michael"); studentDao.updateStudent(student); //get the student studentDao.getStudent(0); System.out.println("Student: [RollNo : " +student.getRollNo()+", Name : "+student.getName()+" ]"); } }
Step 5
Verify the output.
Student: [RollNo : 0, Name : Robert ] Student: [RollNo : 1, Name : John ] Student: Roll No 0, updated in the database Student: [RollNo : 0, Name : Michael ]
Front Controller Pattern
The front controller design pattern is used to provide a centralized request handling mechanism so that all requests will be handled by a single handler. This handler can do the authentication/ authorization/ logging or tracking of request and then pass the requests to corresponding handlers. Following are the entities of this type of design pattern.
- Front Controller - Single handler for all kind of request coming to the application (either web based/ desktop based).
- Dispatcher - Front Controller may use a dispatcher object which can dispatch the request to corresponding specific handler.
- View - Views are the object for which the requests are made.
Implementation
We're going to create a FrontController,Dispatcher to act as Front Controller and Dispatcher correspondingly. HomeView and StudentView represent various views for which requests can come to front controller.
FrontControllerPatternDemo, our demo class will use FrontController ato demonstrate Front Controller Design Pattern.
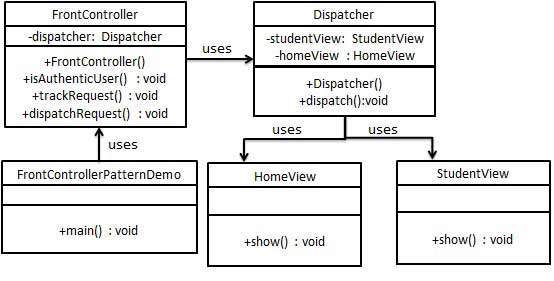
Step 1
Create Views.
HomeView.java
public class HomeView { public void show(){ System.out.println("Displaying Home Page"); } }
StudentView.java
public class StudentView { public void show(){ System.out.println("Displaying Student Page"); } }
Step 2
Create Dispatcher.
Dispatcher.java
public class Dispatcher { private StudentView studentView; private HomeView homeView; public Dispatcher(){ studentView = new StudentView(); homeView = new HomeView(); } public void dispatch(String request){ if(request.equalsIgnoreCase("STUDENT")){ studentView.show(); }else{ homeView.show(); } } }
Step 3
Create FrontController
Context.java
public class FrontController { private Dispatcher dispatcher; public FrontController(){ dispatcher = new Dispatcher(); } private boolean isAuthenticUser(){ System.out.println("User is authenticated successfully."); return true; } private void trackRequest(String request){ System.out.println("Page requested: " + request); } public void dispatchRequest(String request){ //log each request trackRequest(request); //authenticate the user if(isAuthenticUser()){ dispatcher.dispatch(request); } } }
Step 4
Use the FrontController to demonstrate Front Controller Design Pattern.
FrontControllerPatternDemo.java
public class FrontControllerPatternDemo { public static void main(String[] args) { FrontController frontController = new FrontController(); frontController.dispatchRequest("HOME"); frontController.dispatchRequest("STUDENT"); } }
Step 5
Verify the output.
Page requested: HOME User is authenticated successfully. Displaying Home Page Page requested: STUDENT User is authenticated successfully. Displaying Student Page
Intercepting Filter Pattern
The intercepting filter design pattern is used when we want to do some pre-processing / post-processing with request or response of the application. Filters are defined and applied on the request before passing the request to actual target application. Filters can do the authentication/ authorization/ logging or tracking of request and then pass the requests to corresponding handlers. Following are the entities of this type of design pattern.
- Filter - Filter which will perform certain task prior or after execution of request by request handler.
- Filter Chain - Filter Chain carries multiple filters and help to execute them in defined order on target.
- Target - Target object is the request handler
- Filter Manager - Filter Manager manages the filters and Filter Chain.
- Client - Client is the object who sends request to the Target object.
Implementation
We're going to create a FilterChain,FilterManager, Target, Client as various objects representing our entities.AuthenticationFilter and DebugFilter represents concrete filters.
InterceptingFilterDemo, our demo class will use Client to demonstrate Intercepting Filter Design Pattern.
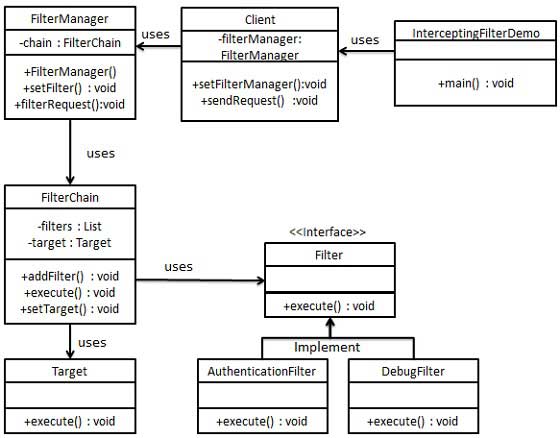
Step 1
Create Filter interface.
Filter.java
public interface Filter { public void execute(String request); }
Step 2
Create concrete filters.
AuthenticationFilter.java
public class AuthenticationFilter implements Filter { public void execute(String request){ System.out.println("Authenticating request: " + request); } }
DebugFilter.java
public class DebugFilter implements Filter { public void execute(String request){ System.out.println("request log: " + request); } }
Step 3
Create Target
Target.java
public class Target { public void execute(String request){ System.out.println("Executing request: " + request); } }
Step 4
Create Filter Chain
FilterChain.java
import java.util.ArrayList; import java.util.List; public class FilterChain { private List<Filter> filters = new ArrayList<Filter>(); private Target target; public void addFilter(Filter filter){ filters.add(filter); } public void execute(String request){ for (Filter filter : filters) { filter.execute(request); } target.execute(request); } public void setTarget(Target target){ this.target = target; } }
Step 5
Create Filter Manager
FilterManager.java
public class FilterManager { FilterChain filterChain; public FilterManager(Target target){ filterChain = new FilterChain(); filterChain.setTarget(target); } public void setFilter(Filter filter){ filterChain.addFilter(filter); } public void filterRequest(String request){ filterChain.execute(request); } }
Step 6
Create Client
Client.java
public class Client { FilterManager filterManager; public void setFilterManager(FilterManager filterManager){ this.filterManager = filterManager; } public void sendRequest(String request){ filterManager.filterRequest(request); } }
Step 7
Use the Client to demonstrate Intercepting Filter Design Pattern.
FrontControllerPatternDemo.java
public class InterceptingFilterDemo { public static void main(String[] args) { FilterManager filterManager = new FilterManager(new Target()); filterManager.setFilter(new AuthenticationFilter()); filterManager.setFilter(new DebugFilter()); Client client = new Client(); client.setFilterManager(filterManager); client.sendRequest("HOME"); } }
Step 8
Verify the output.
Authenticating request: HOME request log: HOME Executing request: HOME
Service Locator Pattern
The service locator design pattern is used when we want to locate various services using JNDI lookup. Considering high cost of looking up JNDI for a service, Service Locator pattern makes use of caching technique. For the first time a service is required, Service Locator looks up in JNDI and caches the service object. Further lookup or same service via Service Locator is done in its cache which improves the performance of application to great extent. Following are the entities of this type of design pattern.
- Service - Actual Service which will process the request. Reference of such service is to be looked upon in JNDI server.
- Context / Initial Context -JNDI Context, carries the reference to service used for lookup purpose.
- Service Locator - Service Locator is a single point of contact to get services by JNDI lookup, caching the services.
- Cache - Cache to store references of services to reuse them
- Client - Client is the object who invokes the services via ServiceLocator.
Implementation
We're going to create a ServiceLocator,InitialContext, Cache, Service as various objects representing our entities.Service1 and Service2 represents concrete services.
ServiceLocatorPatternDemo, our demo class is acting as a client here and will use ServiceLocator to demonstrate Service Locator Design Pattern.
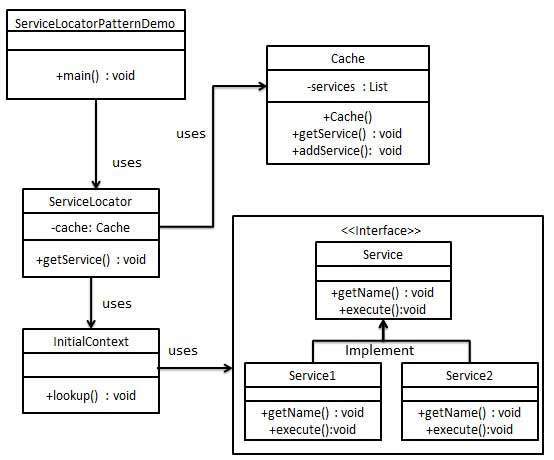
Step 1
Create Service interface.
Service.java
public interface Service { public String getName(); public void execute(); }
Step 2
Create concrete services.
Service1.java
public class Service1 implements Service { public void execute(){ System.out.println("Executing Service1"); } @Override public String getName() { return "Service1"; } }
Service2.java
public class Service2 implements Service { public void execute(){ System.out.println("Executing Service2"); } @Override public String getName() { return "Service2"; } }
Step 3
Create InitialContext for JNDI lookup
InitialContext.java
public class InitialContext { public Object lookup(String jndiName){ if(jndiName.equalsIgnoreCase("SERVICE1")){ System.out.println("Looking up and creating a new Service1 object"); return new Service1(); }else if (jndiName.equalsIgnoreCase("SERVICE2")){ System.out.println("Looking up and creating a new Service2 object"); return new Service2(); } return null; } }
Step 4
Create Cache
Cache.java
import java.util.ArrayList; import java.util.List; public class Cache { private List<Service> services; public Cache(){ services = new ArrayList<Service>(); } public Service getService(String serviceName){ for (Service service : services) { if(service.getName().equalsIgnoreCase(serviceName)){ System.out.println("Returning cached "+serviceName+" object"); return service; } } return null; } public void addService(Service newService){ boolean exists = false; for (Service service : services) { if(service.getName().equalsIgnoreCase(newService.getName())){ exists = true; } } if(!exists){ services.add(newService); } } }
Step 5
Create Service Locator
ServiceLocator.java
public class ServiceLocator { private static Cache cache; static { cache = new Cache(); } public static Service getService(String jndiName){ Service service = cache.getService(jndiName); if(service != null){ return service; } InitialContext context = new InitialContext(); Service service1 = (Service)context.lookup(jndiName); cache.addService(service1); return service1; } }
Step 6
Use the ServiceLocator to demonstrate Service Locator Design Pattern.
ServiceLocatorPatternDemo.java
public class ServiceLocatorPatternDemo { public static void main(String[] args) { Service service = ServiceLocator.getService("Service1"); service.execute(); service = ServiceLocator.getService("Service2"); service.execute(); service = ServiceLocator.getService("Service1"); service.execute(); service = ServiceLocator.getService("Service2"); service.execute(); } }
Step 7
Verify the output.
Looking up and creating a new Service1 object Executing Service1 Looking up and creating a new Service2 object Executing Service2 Returning cached Service1 object Executing Service1 Returning cached Service2 object Executing Service2
Transfer Object Pattern
The Transfer Object pattern is used when we want to pass data with multiple attributes in one shot from client to server. Transfer object is also known as Value Object. Transfer Object is a simple POJO class having getter/setter methods and is serializable so that it can be transferred over the network. It do not have any behavior. Server Side business class normally fetches data from the database and fills the POJO and send it to the client or pass it by value. For client, transfer object is read-only. Client can create its own transfer object and pass it to server to update values in database in one shot. Following are the entities of this type of design pattern.
- Business Object - Business Service which fills the Transfer Object with data.
- Transfer Object -Simple POJO, having methods to set/get attributes only.
- Client - Client either requests or sends the Transfer Object to Business Object.
Implementation
We're going to create a StudentBO as Business Object,Student as Transfer Object representing our entities.
TransferObjectPatternDemo, our demo class is acting as a client here and will use StudentBO andStudent to demonstrate Transfer Object Design Pattern.
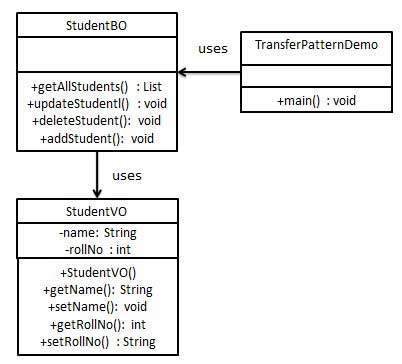
Step 1
Create Transfer Object.
StudentVO.java
public class StudentVO { private String name; private int rollNo; StudentVO(String name, int rollNo){ this.name = name; this.rollNo = rollNo; } public String getName() { return name; } public void setName(String name) { this.name = name; } public int getRollNo() { return rollNo; } public void setRollNo(int rollNo) { this.rollNo = rollNo; } }
Step 2
Create Business Object.
StudentBO.java
import java.util.ArrayList; import java.util.List; public class StudentBO { //list is working as a database List<StudentVO> students; public StudentBO(){ students = new ArrayList<StudentVO>(); StudentVO student1 = new StudentVO("Robert",0); StudentVO student2 = new StudentVO("John",1); students.add(student1); students.add(student2); } public void deleteStudent(StudentVO student) { students.remove(student.getRollNo()); System.out.println("Student: Roll No " + student.getRollNo() +", deleted from database"); } //retrive list of students from the database public List<StudentVO> getAllStudents() { return students; } public StudentVO getStudent(int rollNo) { return students.get(rollNo); } public void updateStudent(StudentVO student) { students.get(student.getRollNo()).setName(student.getName()); System.out.println("Student: Roll No " + student.getRollNo() +", updated in the database"); } }
Step 3
Use the StudentBO to demonstrate Transfer Object Design Pattern.
TransferObjectPatternDemo.java
public class TransferObjectPatternDemo { public static void main(String[] args) { StudentBO studentBusinessObject = new StudentBO(); //print all students for (StudentVO student : studentBusinessObject.getAllStudents()) { System.out.println("Student: [RollNo : " +student.getRollNo()+", Name : "+student.getName()+" ]"); } //update student StudentVO student =studentBusinessObject.getAllStudents().get(0); student.setName("Michael"); studentBusinessObject.updateStudent(student); //get the student studentBusinessObject.getStudent(0); System.out.println("Student: [RollNo : " +student.getRollNo()+", Name : "+student.getName()+" ]"); } }
Step 4
Verify the output.
Student: [RollNo : 0, Name : Robert ] Student: [RollNo : 1, Name : John ] Student: Roll No 0, updated in the database Student: [RollNo : 0, Name : Michael ]
No comments:
Post a Comment